Command pattern က behavioral design pattern တစ်ခုပါ။
Text-Editor app တစ်ခု ဖန်တီးသည့် အခါမှာ toolbar မှာ buttons တွေ အများကြီး ရှိပါ မယ်။ Copy, Paste, Undo, Redo စသည့် button တွေ အများကြီး ရှိပါတယ်။
Save လုပ်ဖို့ အတွက် Button ကနေ ရှိနိုင်တယ်။ Menu ကနေ ရှိနိုင်တယ်။ Shortcut ကနေလည်း ရှိနိုင်ပါတယ်။
Button တစ်ခု ဆီကနေ function ခေါ်မယ့် စား SaveCommand ဆိုပြီး command pattern နဲ့ ရေးတာ ပိုအဆင်ပြေပါမယ်။
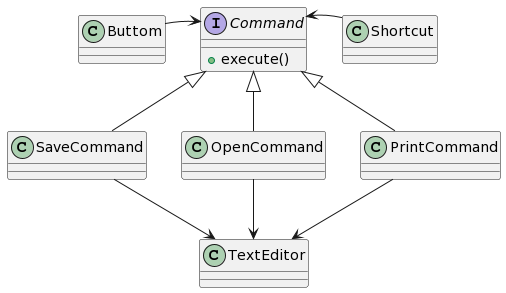
ပုံမှာ ဆိုရင် Button နဲ့ Shortcut က Command Interface ကနေ တဆင့် execute လုပ်တော့မယ့် အပိုင်းပဲ ရှိပါတော့တယ်။ Command တိုင်းမှာ execute function ပါပြီး သက်ဆိုင်ရာ Function ကို execute လုပ်သွားမှာပါ။
Java code ကို ကြည့်ရအောင်။
Command.java
public interface Command {
void execute();
}
SaveCommand.java
public class SaveCommand implements Command {
private TextEditor textEditor;
public SaveCommand(TextEditor textEditor) {
this.textEditor = textEditor;
}
@Override
public void execute() {
textEditor.save();
}
}
OpenCommand.java
public class OpenCommand implements Command {
private TextEditor textEditor;
public OpenCommand(TextEditor textEditor) {
this.textEditor = textEditor;
}
@Override
public void execute() {
textEditor.open();
}
}
PrintCommand.java
public class PrintCommand implements Command {
private TextEditor textEditor;
public PrintCommand(TextEditor textEditor) {
this.textEditor = textEditor;
}
@Override
public void execute() {
textEditor.print();
}
}
TextEditor.java
public class TextEditor {
public void save() {
System.out.println("Saving the document.");
// Save implementation here
}
public void open() {
System.out.println("Opening a document.");
// Open implementation here
}
public void print() {
System.out.println("Printing the document.");
// Print implementation here
}
}
Button.java
public class Button {
private Command command;
public Button(Command command) {
this.command = command;
}
public void click() {
command.execute();
}
}
Shortcut.java
public class Shortcut {
private Command command;
public Shortcut(Command command) {
this.command = command;
}
public void press() {
command.execute();
}
}
Client.java
public class Client {
public static void main(String[] args) {
TextEditor textEditor = new TextEditor();
// Create command objects and associate them with the receiver
Command saveCommand = new SaveCommand(textEditor);
Command openCommand = new OpenCommand(textEditor);
Command printCommand = new PrintCommand(textEditor);
// Create sender objects (buttons or shortcuts)
Button saveButton = new Button(saveCommand);
Button openButton = new Button(openCommand);
Shortcut printShortcut = new Shortcut(printCommand);
// Simulate the user clicking the buttons or using shortcuts
saveButton.click();
openButton.click();
printShortcut.press();
}
}
ဒီ Code လေးကို ကြည့်လိုက်ရင် သဘောပေါက်လွယ်မှာပါ။
Command Pattern မှာ
- Invoker
- Command
- Concrete Command
- Reciver
ဆိုပြီး ရှိပါတယ်။
Button, Short တို့က Invoker
ပါ။ သူတို့က command ကို invoke လုပ်မယ့်သူတွေပါ။
Command interface ကတော့ Command
ဖြစ်ပြီး execute လုပ်မယ့် အပိုင်းပဲ ပါဝင်ပါတယ်။
SaveCommand, PrintCommand, OpenCommand တွေကတော့ Concrete Command
ပါ။
TextEditor ကတော့ Receiver
ပါ။ Command တွေက Reciver ရဲ့ operation တွေကို လှမ်းခေါ်မှာပါ။
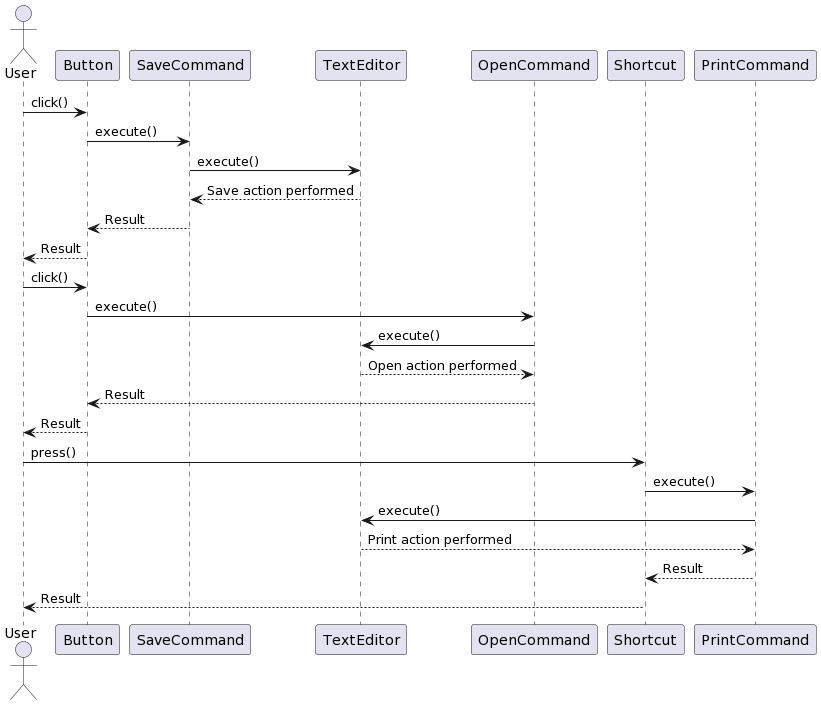
Pros and Cons
ကောင်းတာတွေကတော့
- Single Responsiblity Principle ကို လိုက်နာ ထားတယ်။
- Open/Close Principle ကိုလည်း လိုက်နာ ပါတယ်။
- Complex ဖြစ်သည့် system အတွက် ရိုးရှင်း Commands တွေ စုစည်းပြီး ရေးသားနိုင်ပါတယ်။ ဥပမာ TextEditor ရဲ့ Toolbar button တွေလိုမျိုးပေါ့။
မကောင်းတာကတော့
- Code က ပိုပြီး complicated ဖြစ်သွားနိုင်တယ်။ Sender နဲ့ Receiver ကြားမှာ နောက်ထပ် layer တစ်ခု ပါလာသလို ဖြစ်သွားတာပါ။