Factory Pattern က Creational Pattern တစ်ခု ဖြစ်ပါတယ်။ Factory ဆိုသည့် အတိုင်း စက်ရုံကနေ ပစ္စည်း ထုတ် သလို object တွေကို ဖန်တီးပေးသည့် pattern တစ်ခုပါ။
Factory pattern မသုံးခင်မှာ Object တွေကို လက်ရှိ code တွေထဲကနေ ရောရေးပြီး ဖန်တီးပါတယ်။ Factory pattern က single responbility ဖြစ်ပြီး separation of concern အရ object ဖန်တီး မှု ကို ခွဲထုတ်ရေးသားလိုက်ခြင်း မျိုးပါပဲ။
Factory Pattern မသုံးခင် java code ကို ကြည့်ရအောင်။
public class Shape {
public void draw() {
System.out.println("Drawing a shape");
}
}
public class Circle extends Shape {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Rectangle extends Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle");
}
}
public class Client {
public static void main(String[] args) {
Shape circle = new Circle();
circle.draw();
Shape rectangle = new Rectangle();
rectangle.draw();
}
}
Client
class က Cirlce နဲ့ Recetangle ကို main class ထဲမှာ ထည့်ရေးထားပါတယ်။
Factory pattern ပြောင်းပြီး ရေးမယ် ဆိုရင်
// Shape interface
public interface Shape {
void draw();
}
// Concrete Shape classes
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle");
}
}
// Factory class
public class ShapeFactory {
public static Shape getShape(String shapeType) {
if (shapeType.equals("circle")) {
return new Circle();
} else if (shapeType.equals("rectangle")) {
return new Rectangle();
} else {
throw new IllegalArgumentException("Invalid shape type");
}
}
}
// Client code
public class Client {
public static void main(String[] args) {
Shape circle = ShapeFactory.getShape("circle");
circle.draw();
Shape rectangle = ShapeFactory.getShape("rectangle");
rectangle.draw();
}
}
Code ကတော့ အတူတူပါပဲ။ ShapeFactory
ကနေ shape တွေ ထုတ်ပေးသည့် သဘောပါ။
Class Diagram ကို ကြည့်ရအောင်။
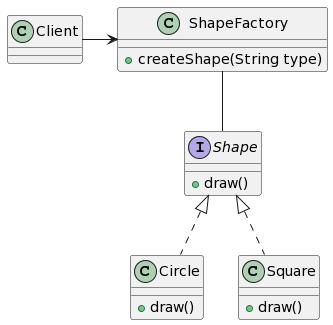
Factory Pattern ကို proxy pattern နဲ့ လည်း တွဲပြီး သုံးကြပါတယ်။
Pros and Cons
Tight coupling မဖြစ်အောင် ရှောင်ရှားနိုင်ပါတယ်။
Single Responsibility နဲ့ Open/Closed Principle ကို လိုက်နာ ထားပါတယ်။
မကောင်းတာကတော့ code တွေ complicated ဖြစ်နိုင်ပါတယ်။ မလိုအပ်ပဲ subclass တွေ များလာနိုင်ပါတယ်။