Map Reduce ကို ပထမဆုံး သိခဲ့တာကတော့ Mongodb မှာပါ။ သို့ပေမယ့် အစိမ်းသပ်သပ်ကြီး ဖြစ်နေသည့် အတွက်ကြောင့် ချက်ခြင်း နားမလည်ခဲ့ဘူး။ နောက်ပြီး ဘယ်လို အသုံးပြုရမလဲဆိုသည့် Logic လည်း မရှိခဲ့ဘူး။
Map Reduce ဆိုပေမယ့် အများအားဖြင့် filter , map , reduce ဆိုပြီး တွဲသုံးကြတာ များပါတယ်။
Map Reduce ကို နားလည်လွယ်ဆုံး ပုံစံကတော့
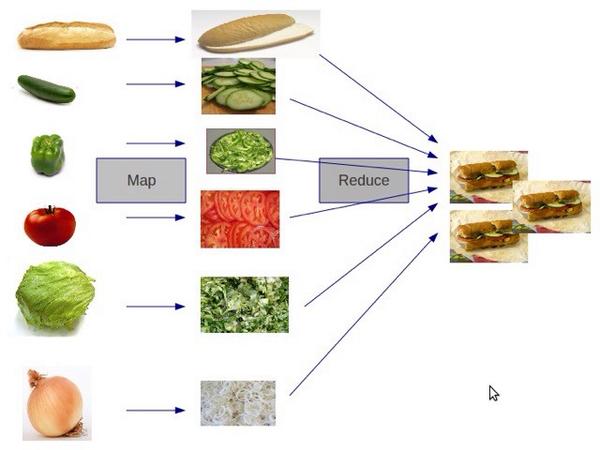
ကျွန်တော်တို့မှာ ရှိသည့် data တွေကို လိုချင်သည့် ပုံစံရအောင် map လုပ်ပါတယ်။ ပြီးရင် data တွေ အကုန်လုံးကို ပေါင်းလိုက်ပြီးတော့ နောက်ဆုံး တကယ်ရလိုသည့် result ကို ထုတ်ပါတယ်။
ကျွန်တော်တို့ ဥပမာလေး တစ်ခုနဲ့ စလိုက်ရအောင်။ Map Reduce ဟာ javascript မှာ ပါလာပြီ ဖြစ်သည့်အတွက် ကျွန်တော် javascript နဲ့ပဲ ဖော်ပြလိုက်ပါမယ်။
class Person {
constructor(name,age) {
this.name = name
this.age = age
}
}
let people = [new Person("Mg Aye",20),new Person("Ko Maung",32),
new Person("Mg Aye",50),new Person("Mg Aye",49),
new Person("Mg Aye",22),new Person("Mg Aye",18)]
အခု ဆိုရင် people ဆိုသည့် array ထဲမှာ Person object တွေရှိပါတယ်။ ကျွန်တော်တို့ လူတွေ အားလုံးရဲ့ စုစုပေါင်း အသက်ကို သိချင်တယ် ဆိုပါစို့။
သမာရိုးကျ စဉ်းစားရင်တော့ array ကို loop ပတ်။ variable တစ်ခုထဲမှာ age ကို ထည့်ပြီး ပေါင်းသွားရုံပါပဲ။
let age = 0;
people.forEach(function (people) {
age = age + people.age
});
console.log(age)
ကျွန်တော်တို့ အနေနဲ့ map reduce သုံးပြီးတော့ အခု လိုရေးလို့ရပါတယ်။
let age = people.map(people => people.age).reduce((prev,age) => prev+age)
console.log(age)
Code က တစ်ကြောင်းတည်း နဲ့ ပြီးသွားပါတယ်။
Map
people.map(people => people.age)
ဆိုတာကတော့ အကျယ်ချဲ့လိုက်ရင်
people.map(function(people) {
return people.age
})
နဲ့ တူပါတယ်။
map ကတော့ array object ကို ကိုလိုချင်သည့် ပုံစံကို ပြောင်းလိုက်တာပါ။
let age_array = people.map(people => people.age)
အဲဒီလိုဆိုရင် age_array
ထဲမှာ [ 20, 32, 50, 49, 22, 18 ]
ဆိုပြီး ဝင်သွားပါမယ်။
Reduce
.reduce((prev,age) => prev+age)
ဆိုတာကို ချဲ့ပြီးရေးလိုက်ရင်
.reduce(function(prev,age) {
return prev+age
})
reduce ကတော့ array ကို နောက်ဆုံး တစ်ခုတည်း ဖြစ်သွားအောင် ဖန်တီးပေးပါတယ်။ prev ကတော့ ကျွန်တော်တို့ return ပြန်လိုက်သည့် data ပေါ့။
ပိုရှင်းသွားအောင်
[ 20, 32, 50, 49, 22, 18 ].reduce(function(prev,age) {
console.log("PREV " + prev)
console.log("AGE " + age)
return prev+age
})
PREV 20
AGE 32
PREV 52
AGE 50
PREV 102
AGE 49
PREV 151
AGE 22
PREV 173
AGE 18
ဆိုပြီး ထွက်လာပါလိမ့်မယ်။
အခုဆိုရင် map reduce ကို မြင်လာပြီ ထင်တယ်။
Filter
အခု filter ကိုပါ တွဲပြီး သုံးကြည့်ရအောင်။
အသက် ၃၀ ကျော်လူတွေရဲ့ အသက်စုစုပေါင်းကို သိချင်တယ်ဆိုပါစို့။
let age = people.filter(people => people.age > 30).map(people => people.age).reduce((prev,age) => prev+age)
console.log(age)
filter က အဲဒီ return true
ပြန်ရင် အဲဒီ အခန်းက value ကို ယူတယ်။ return false
ပြန်ရင် အဲဒီ အခန်းက value ကို ignore လုပ်သွားပါတယ်။ people.age > 30
ဆိုတော့ 30 ထက် ကျော်သည့်သူတွေကိုသာ return လုပ်ပေးသွားမှာပါ။
filter ကိုတော့ ကျွန်တော် အကျယ် မရှင်းပြတော့ပါဘူး။ အထက်က map reduce နဲ့ ဆင်ပါတယ်။
code ကို နည်းနည်းရှင်းအောင် တစ်ခါထက် မနည်း ခေါ်နိုင်အောင် အောက်ကလို function ခွဲထုတ်ပြီးလည်း အသုံးပြုနိုင်ပါတယ်။
let max_age = (people,max) => people.age > max
let total_age = (people,age) => people.filter(people => max_age(people,age)).map(people => people.age).reduce((prev,age) => prev+age)
let over_age_30 = total_age(people,30)
let over_age_20 = total_age(people,20)
console.log(over_age_30)
console.log(over_age_20)
Filter, Map , Reduce
Filter, Map, Reduce စတာတွေ ရှိလာသည့် အခါမှာ အချို့ စဉ်းစားပုံလေးတွေ အနည်းငယ် ကွာသွားတာကို တွေ့နိုင်ပါတယ်။ ကျွန်တော်တို့တွေ အနေနဲ့ loop တွေ အများကြီး ပတ်ပြီးတော့ တစ်ဆင့်ပြီး တစ်ဆင့်အစား Filter, Map, Reduce ကို သုံးပြီးတော့ အဆင့်တွေကို လျော့ချနိုင်လာပါတယ်။
Leave a Reply