Template ဆိုတာကတော့ ကျွန်တော်တို့တွေ ကြားနေကြပါ။ ပုံမှန် အားဖြင့် အရန်သင့်ရှိပြီးသားထဲကမှ လိုအပ်သည့် အပိုင်းလေးကိုပဲ ပြင်ပြီး သုံးတာပါ။
Template Method Pattern ကလည်း အဲလိုခပ်ဆင်ဆင်ပါပဲ။ Template Method Pattern က Behavioral Patterns တစ်ခုပါ။
Class Diagram ကို ကြည့်ရအောင်။
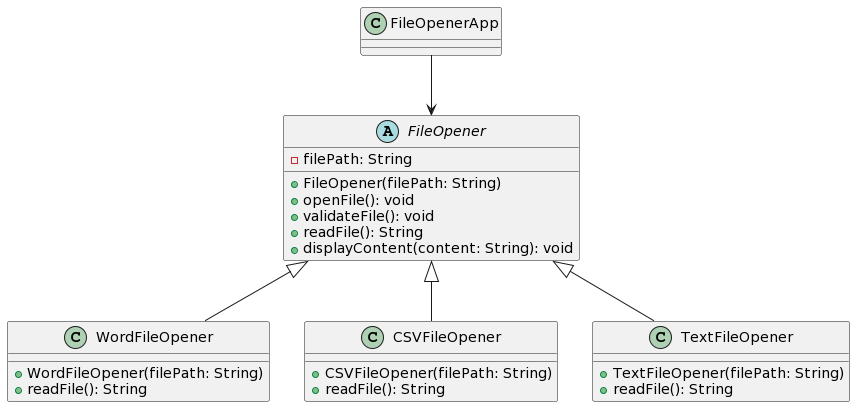
Java code က
abstract class FileOpener {
private String filePath;
public FileOpener(String filePath) {
this.filePath = filePath;
}
// Template method that defines the steps for opening the file
public final void openFile() {
validateFile();
String content = readFile();
displayContent(content);
}
// Common methods shared by all file types
private void validateFile() {
// Implement file validation logic here
System.out.println("Validating file: " + filePath);
}
// Abstract method that subclasses need to implement
protected abstract String readFile();
void displayContent(String content) {
System.out.println("Displaying file content: \n" + content);
}
}
class WordFileOpener extends FileOpener {
public WordFileOpener(String filePath) {
super(filePath);
}
@Override
protected String readFile() {
// Implement logic to read a Word document (.docx) file
System.out.println("Reading Word file: " + getFilePath());
return "Content of the Word file";
}
}
class CSVFileOpener extends FileOpener {
public CSVFileOpener(String filePath) {
super(filePath);
}
@Override
protected String readFile() {
// Implement logic to read a CSV file
System.out.println("Reading CSV file: " + getFilePath());
return "Content of the CSV file";
}
}
class TextFileOpener extends FileOpener {
public TextFileOpener(String filePath) {
super(filePath);
}
@Override
protected String readFile() {
// Implement logic to read a plain text file
System.out.println("Reading text file: " + getFilePath());
return "Content of the text file";
}
}
public class FileOpenerApp {
public static void main(String[] args) {
String wordFilePath = "path/to/word/document.docx";
String csvFilePath = "path/to/csv/file.csv";
String textFilePath = "path/to/text/document.txt";
FileOpener wordFileOpener = new WordFileOpener(wordFilePath);
FileOpener csvFileOpener = new CSVFileOpener(csvFilePath);
FileOpener textFileOpener = new TextFileOpener(textFilePath);
System.out.println("Opening Word file...");
wordFileOpener.openFile();
System.out.println("\nOpening CSV file...");
csvFileOpener.openFile();
System.out.println("\nOpening text file...");
textFileOpener.openFile();
}
}
FileOpner က File ဖတ်သည့် အခါမှာ validate လုပ်တယ်။ ပြီးရင် File Open လုပ်တယ်။ ပြီးရင် readFle လုပ်ပြီး display ပြပေးတယ်။ CSV, Text,Doc တွေမှာ အခြား အဆင့်တွေ အကုန်တူပြီး readfile တစ်ခုတည်း မတူတာပါ။ step တွေ တူပြီး implentation မတူသည့် အခါမှာ Template Pattern က သင့်တော်ပါတယ်။
Template Pattern က web framework တွေမှာ view အတွက် သုံးတာတွေ ကို တွေ့နိုင်တယ်။ view မှာ Header Footer တူပြီး body မတူသည့် အခါမှာ template ခွဲရေးသည့် ပုံစံ နဲ့ တူပါတယ်။
Pros and Cons
Step တူပြီး Implemention မတူသည့် အခါမှာ template pattern ကို အသုံးပြုနိုင်ပါတယ်။ Code တွေကို ပိုလိုရှင်းလင်း စေပါတယ်။ နောက်ပြီး algorithm တစ်ခုကနေ နောက်တစ်ခုကို ပြောင်းလဲ နိုင်ပါတယ်။
Superclass က code ကိုလည်း ပြန်ပြီး အသုံးပြုနိုင်ပါတယ်။
မကောင်းတာကတော့ Liskov Substitution Principle ကို ချိုးဖောက်နိုင်ပါတယ်။ တချို့ sub class တွေက မလိုသည့် အဆင့်တွေ ပါလာနိုင်ပါတယ်။
step တွေ များလာလေလေ maintain လုပ်ဖို့ ခက်လေလေပါပဲ။