State Pattern ကတော့ Behaviour Pattern တစ်ခုပါ။ State Pattern ကတော့ strategy pattern နဲ့ တူသလို နဲ့ မှားတတ်ပါတယ်။ State Pattern ကတော့ strategy pattern လိုပါပဲ။ ဒါပေမယ့် သူက state တစ်ခု ကနေ တစ်ခု ပြောင်းလဲ သွားတာ ရှိပါတယ်။
ဥပမာ။ Level 1 မှာ play 1 point ရမယ်။ Level 1 မှာ point 100 ရရင် Level 2 ကို ပြောင်းမယ်။ Level 2 မှာ play တစ်ခါ လုပ်တိုင်း 2 point ရမယ်။ Level 3 ကို point 300 ရရင် ပြောင်းသွားမယ်။ Level 3 မှ play တစ်ခါလုပ်တိုင်း 3 point မယ်။
သူရဲ့ State Diagram လေးက အောက်ကလိုပါ။
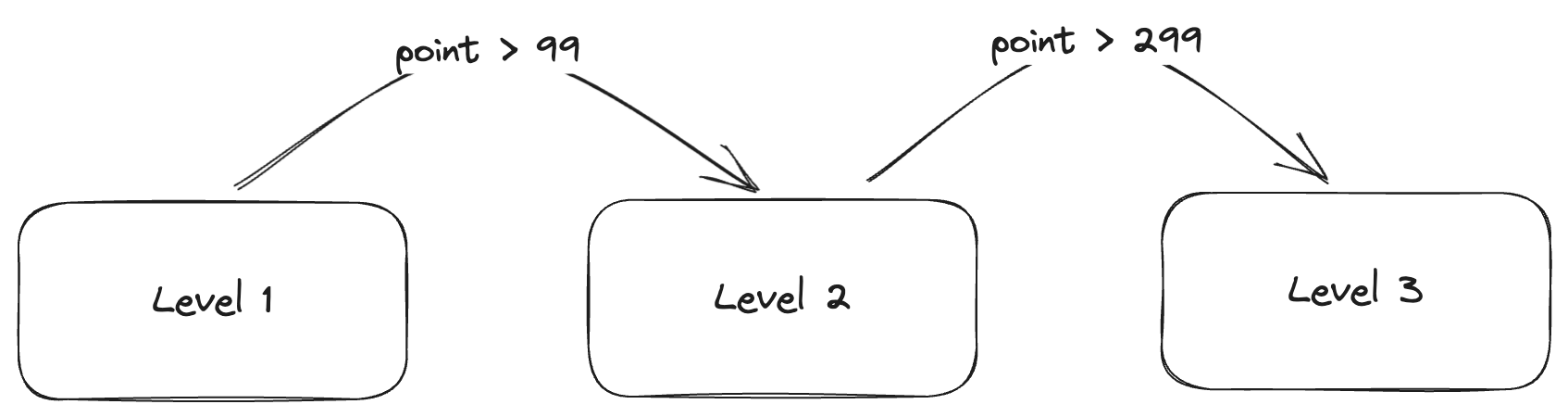
ပုံမှန် အားဖြင့် ရေးကြရင်
//Game.java for Game Class
if (level == 1) {
point = point + 1;
}
else if (level == 2) {
point = point + 2;
}
else if (level == 3) {
point = point + 3;
}
if (point > 99) {
level = 2;
}
else if (point > 299) {
level = 3;
}
Level 4 ထပ်ဖြည့်ရင် နောက်တစ်ခါ ထပ်ပြီး ပြင်ရပါအုံးမယ်။
ဒါကြောင့် state pattern ကို ပြောင်းသုံးပြီး ပြင်ပါမယ်။
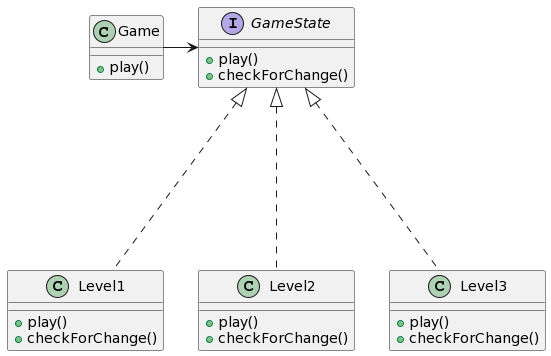
Java code ကို ကြည့်ရအောင်။
public interface GameState {
void play();
void checkForChange();
}
public class Game {
private GameState currentState;
private int totalPoint;
public Game() {
this.totalPoint = 0;
}
public void setState(GameState state) {
if (state != null) {
currentState = state;
}
}
public void play() {
if (currentState != null) {
currentState.play();
currentState.checkForChange();
}
}
public int getTotalPoint() {
return totalPoint;
}
}
public class Level1 implements GameState {
private final Game game;
Level1(Game game) {
this.game = game;
}
@Override
public void play() {
game.totalPoint += 1;
}
@Override
public void checkForChange() {
if (game.totalPoint > 99) {
Level2 lvl2 = new Level2(game);
game.setState(lvl2);
}
}
}
public class Level2 implements GameState {
private final Game game;
Level2(Game game) {
this.game = game;
}
@Override
public void play() {
game.totalPoint += 2;
}
@Override
public void checkForChange() {
if (game.totalPoint > 299) {
Level3 lvl3 = new Level3(game);
game.setState(lvl3);
}
}
}
public class Level3 implements GameState {
private final Game game;
Level3(Game game) {
this.game = game;
}
@Override
public void play() {
game.totalPoint += 3;
}
@Override
public void checkForChange() {
//add codes
}
}
Pros and Cons
Single Responsibility ကို လိုက်နာထားပါတယ်။ State တစ်ခုဟာ သူ့ သက်ဆိုင်ရာ အလုပ်ပဲ လုပ်ပါတယ်။
Open/Closed Principle ကို လိုက်နာထားပါတယ်။ State တစ်ခု ကို ထပ်ဖြည့်ဖို့ လက်ရှိ code အဟောင်းကို ပြင်ဖို့ မလိုပါဘူး။
မကောင်းတာကတော့ state နည်းနည်းလေးပဲ ရှိသည့် အခါမှာတော့ over kill ဖြစ်နိုင်ပါတယ်။